This is dramatization conversation between 3 people about IoC.
Layman - Srikanth, what does IoC mean to me.Srikanth - Imagine “You are very lazy man, But you still want to eat and knows what to eat. And you wish when ever you are hungry there was some magic that will prepare and give what you want.
You do not want to prepare, maintain and clean your dishes and you can still eat what ever you want.”
Layman – wow, that’s great, I really wish something like this happen. Alright that’s all I would like to know about, Hey this is my friend JavaMan, I do not know, why this guy keeps looking for new things and keeps reading about new things, I wish he has life. I have to play golf, I would like to leave.
You both can continue.
JavaMan – Hey, what’s up. So tell me more about the IoC man.
Srikanth - IoC known as Inversion of Control (IoC) pattern, also known as Dependency Injection had been around for years but became popular recently especially with advent of Spring framework while developing light weight J2EE container.
JavaMan – Ok, can you tell me in terms of classes, what does it mean ?
Srikanth – Sure, In simple words "Do not use new() in your code for creating your Application Objects".
And Lets take an example Student and Student's Course.
Imagine Class Student has a relationship with Class Course: it wants to use the services of Class Course. The usual way to establish this relationship is to instantiate Class Course inside Class Student.
public class Student {
private Course studentCourse;
public Student() {
studentCourse = new Course();
}
}
Alternatively, if you use Spring Framework's IoC pattern, the responsibility of creating Object "studentCourse" moves from Class "Student" to the IoC framework, which creates and injects Object "studentCourse" into Object "Student ".
public class Student {
private Course studentCourse;
public Student() {
}
public setCourse(Course studentCourse) {
this.studentCourse = studentCourse;
}
}
Srikanth – Imagine the changes in Course, imagine the changes in constructor for example, instead of constructor without parameter, you have constructor with parameters. You have to change you Student class again.
Imagine this change in large enterprise web application. More over you gain the control from one place the creation of all the objects.
And there are more things you can do, we will talk about those soon.
Did you get the concept of IoC ?
JavaMan – I think so, I got little bit, I think I have to get my hands dirty now with this concept. How about the famous example “HelloWorld”. Can you tell me how to write HelloWorld Example with this.
Srikanth – Sure, Ok fire up your famous editor. I have eclipse and I will use that.
Drop some of the Jars we require for this to work. (standard.jar, spring.jar, commons-logging.jar)
Create a Java Project and then create a package “com.atasisoft.project.HelloWorld”
Alright now you are ready to start the code.
First thing first, start with interface.
package com.atasisoft.project.HelloWorld;
public interface HelloWorldService {
public void sayHelloWorld();
}
Next, write the implementation class for the above interface.
package com.atasisoft.project.HelloWorld;
public class HelloWorldServiceImpl implements HelloWorldService {
public String helloWorldMessage;
public HelloWorldServiceImpl() {
}
public HelloWorldServiceImpl(String helloWorldMessage) {
this.helloWorldMessage = helloWorldMessage;
}
public String gethelloWorldMessage() {
return helloWorldMessage;
}
public void sethelloWorldMessage(String helloWorldMessage) {
this.helloWorldMessage = helloWorldMessage;
}
public void sayHelloWorld() {
System.out.println(helloWorldMessage);
}
}
Most of it is done, we have written interface and we have written class that implements interface. We just need the calling class and magic thing of IoC, which will create and maintain our Application Objects for us.
package com.atasisoft.project.HelloWorld;
import org.springframework.beans.factory.BeanFactory;
import org.springframework.beans.factory.xml.XmlBeanFactory;
import org.springframework.core.io.ClassPathResource;
public class HelloApp {
public static void main(String arg[]) throws Exception {
BeanFactory factory = new XmlBeanFactory(new ClassPathResource(
"hello.xml"));
HelloWorldService helloWorldService = (HelloWorldService) factory
.getBean("helloWorldService");
helloWorldService.sayHelloWorld();
}
}
Almost done, all you need the magic file(Hello.xml), keep in the classpath (Since we are using ClassPathResource to find our Hello.xml ).
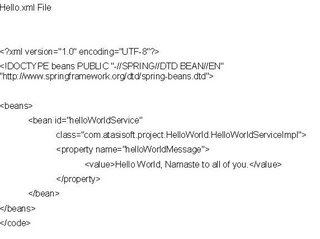
All done!. If you are using Eclipse IDE the workspace will look something like this.
Run the HelloApp class and you will see your results.
Hope you got it all working.
JavaMan - So, what did we gain here.
Srikanth - good question, well with help of Spring framework we are able to manage and configure our application objects.
We are at best in Object Oriented design, our objects are loosely coupled.
This is just a start, using this building blocks and using other features like AOP on top of IoC, we can design best Enterprise Application.
Notes:-
1. All Spring Beans are Singletons.
2. The above example is setter Injection, you need to have setter method for the above example to work. Apart from setter injection, you can also use constructor Injection.
3. Autowiring - you can wire (autowire) your bean in configuration file. There are 4 types of autowiring - byName, byType, constructor, autodetect.
Next topic we will talk about is AOP.
11 comments:
nice explanation ! Thanx
Cool..
Could you talk about wiring concept..
willing to hear from you :-)
Really really great, thanks for this article
Hi Srikant Koppisetti,
Nice Example and explantion
Hema Gubbala
Hi Srikant Koppisetti,
Nice Example and explantion
Hema Gubbala
Great work thanx
may i know what is "traditional interaction mode"..
can u help me in this!
thank you sir
nice explanation
nice explanation. Thanks
nice explaination
Post a Comment